How to Make a Responsive Bootstrap Navbar
Bootstrap provides several standard components to make creating web layouts easier. Just about every web site has a navigation bar and Bootstrap has a navbar component that makes styling responsive site navigation easier.
Navbars are specialized containers for site navigation. They collapse in small viewports and become horizontal as space is available. Bootstrap has been designed to allow you to include non-menu navigational items, like site logos and form elements.
For this tutorial I will use a personal site, SpartanObstacles.com I am building as an example. It won't use all the Bootstrap features in production, but it will allow me the ability to play around to demonstrate features.
You can find example source code in the samples GitHub repository
The Spartan Obstacles navbar Requirements
Before writing code let's define some requirements:
- Site logo in the top left corner
- Site logo is a hot link to the site's home page
- Site menu should render centered (text), but left aligned in relation to the page (to the right of the logo)
- A site search should render on the right side of the header with white space between menu and search form
- The menu should be responsive, hide on smaller screens
- A menu button should display on the left side on smaller view ports and trigger the menu display
- Any sub menus should display when the parent menu item is selected
The Skeleton Bootstrap Navbar HTML
To get the process started I defined wrote some markup and applied the Bootstrap classes needed to get a basic navbar started.
Bootstrap Navbar Components
Bootstrap provides a series of classes to make a navbar responsive. This is a quick list of classes and their purpose plus some general navbar guidance:
- .navbar with .navbar-expand{-sm|-md|-lg|-xl} wrap the entire navbar for responsive collapsing and color scheme classes.
- Navbars and their contents are fluid by default. Use optional containers to limit their horizontal width.
- Use the Bootstrap spacing and flex utility classes for controlling spacing and alignment within navbars.
- Navbars are responsive by default, but you can easily modify them to change that. Responsive behavior depends on our Collapse JavaScript plugin, but that can easily be replaced with a few lines of JavaScript.
- Navbars are hidden when printing. You can force them to be printed by adding .d-print to the .navbar.
- Ensure accessibility by using a
Bootstrap includes additional navigation classes to manage 'sub-content'. These are components that might be part of your site's navigation, but may not be part of the primary menu or provide user interaction points.
- navbar-brand for your company, product, or project name. Makes the element display inline-block and adds some padding, margin and font-size.
- navbar-logo sets the image's width to 15vw or 15% of the viewport's width.
- navbar-nav for a full-height and lightweight navigation (including support for dropdowns).
- navbar-toggler & navbar-toggler-icon classes are for use with our collapse plugin and other navigation toggling behaviors.
- form-inline for any form controls and actions.
- navbar-nav contains the menu. This will typically be an UL.
- navbar-text for adding vertically centered strings of text.
- collapse.navbar-collapse for grouping and hiding navbar contents by a parent breakpoint.
The example navbar includes the Spartan Obstacles logo with a top level menu to the site's main pages. Everything is left aligned.
If the site is loaded on a phone, smaller view port, the menu is hidden and the hamburger menu is displayed.
The navbar-light class apply some default colors to the navbar. For example the nav-items text is automatically styled to use black and initial font-size and item padding.
As the tutorial progresses I will adjust the menu item style so it does not look so default.
Toggling A Mobile Menu
If you want to use the Bootstrap jQuery plugins you need to include jQuery and of course the component script. This can severely affect your page load time, especially on mobile.
The Bootstrap collapse plugin is what toggles the menu expansion and collapse on smaller view ports.
Fortunately that can easily be implemented in 3 lines of JavaScript.
document.querySelector(".navbar-toggler").addEventListener("click", function(e){ document.querySelector("#SpartanNavbar").classList.toggle("show"); });
The toggle button (hamburger icon is standard) has the class 'navbar-toggle'. You can use this as a selector.
Get a reference to the button and add a click event handler.
Inside the click event handler select the menu container, '#SpartanNavbar' and toggle the 'show' class.
This can easily be done using the menu's classList.toggle method.
Now each time the menu toggle button is clicked the show class will be added or removed from the menu element.
There are many opportunities like this, where you can replace large bodies of code with just a few lines if you use native APIs.
Wrapping Things Up
At this point the site has a simple navigation header with menu. It contains a logo and menu. The menu collapses so it is hidden on smaller screens.
A toggle button is visible when it is collapsed. At this point it is not wired up, so the menu is not accessible.
I will continue to update this article with more details over the next few days. :)
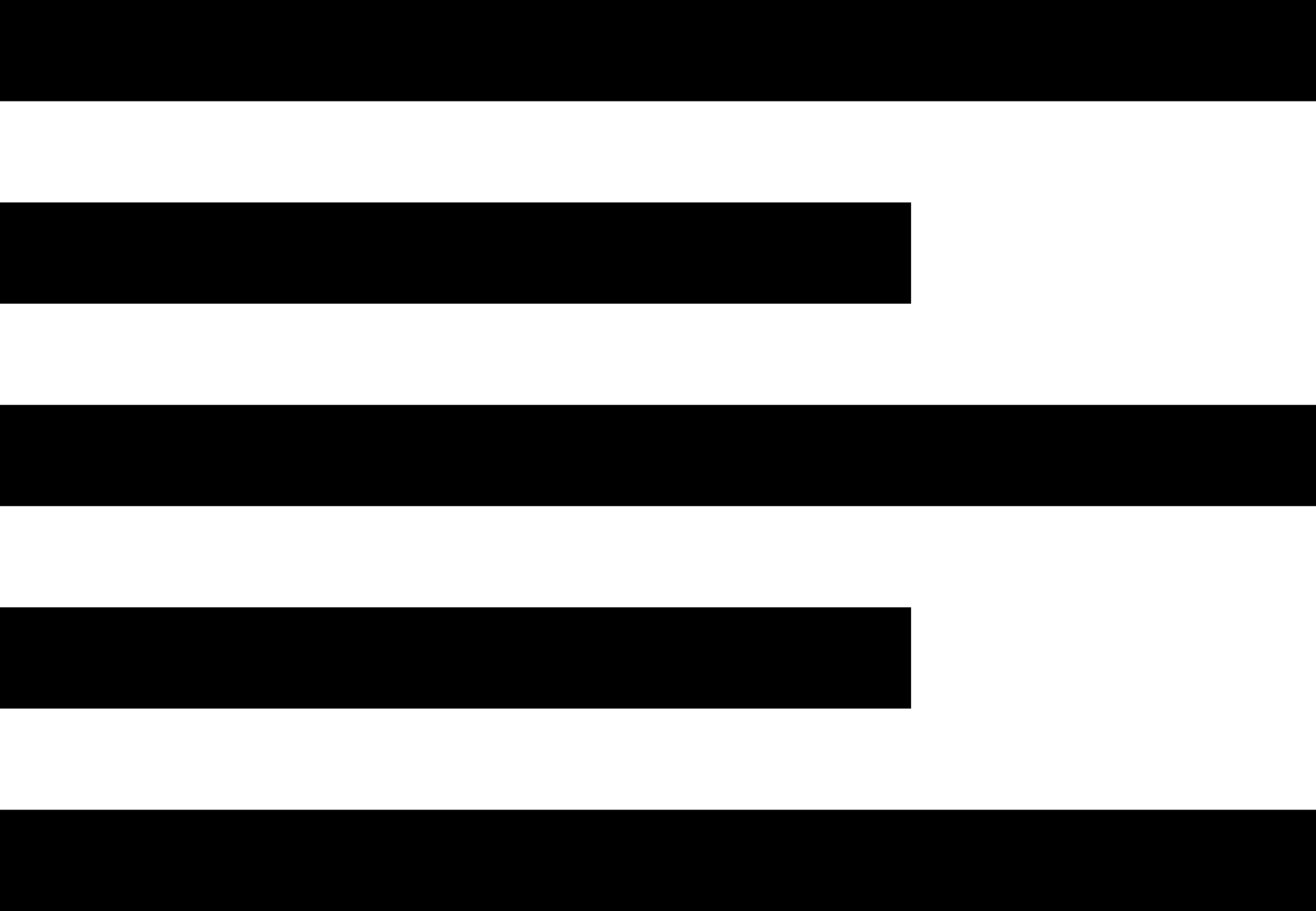
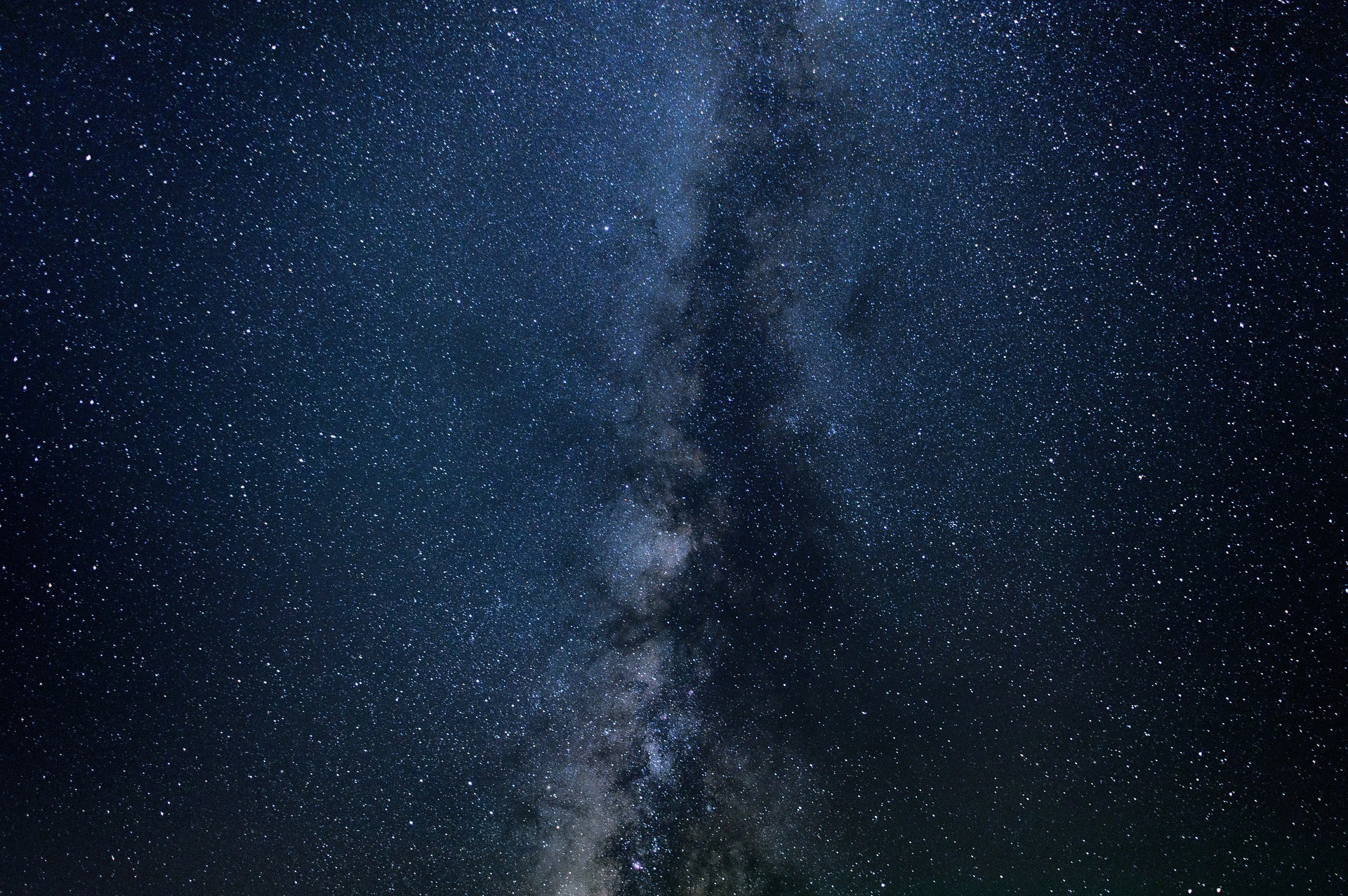
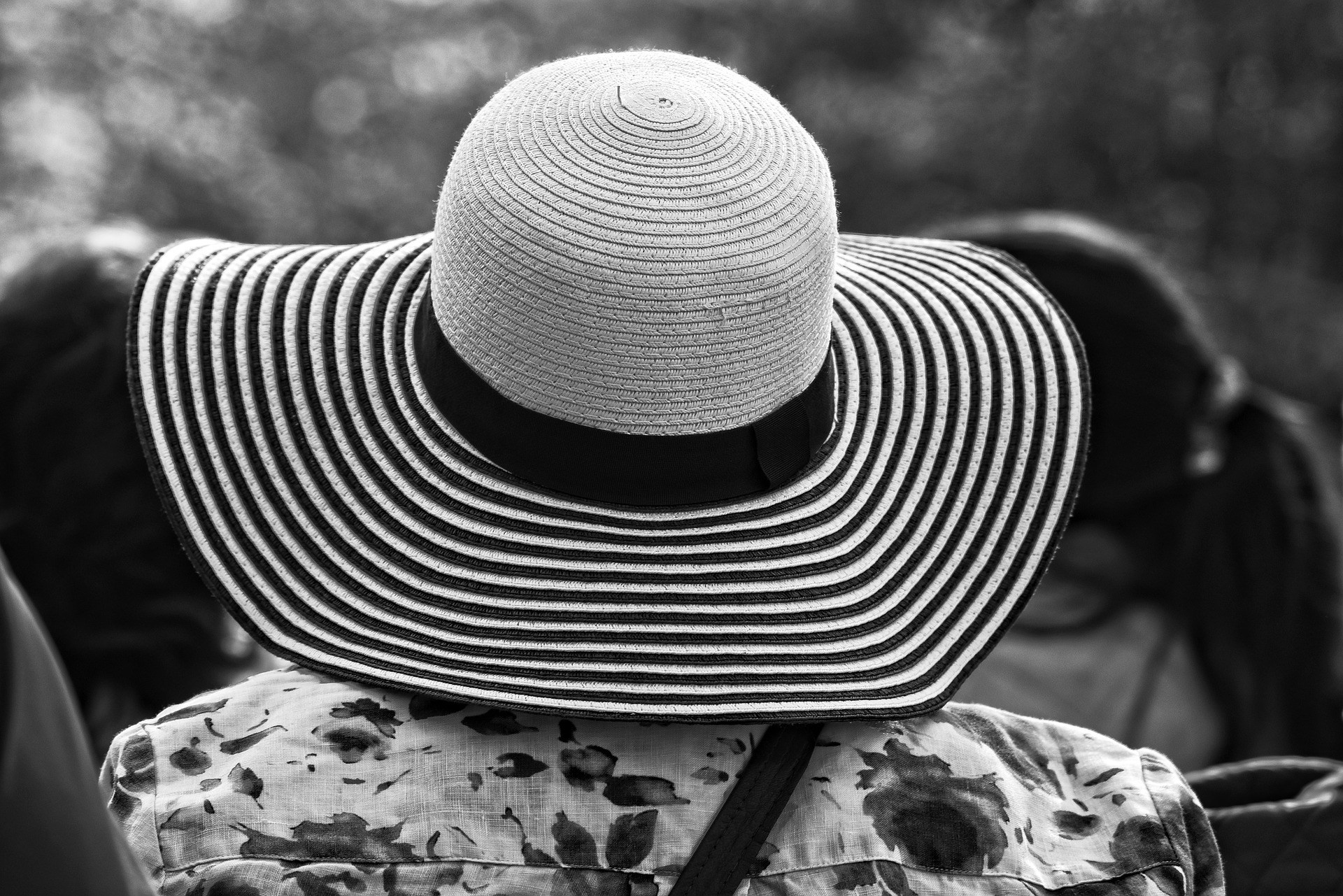
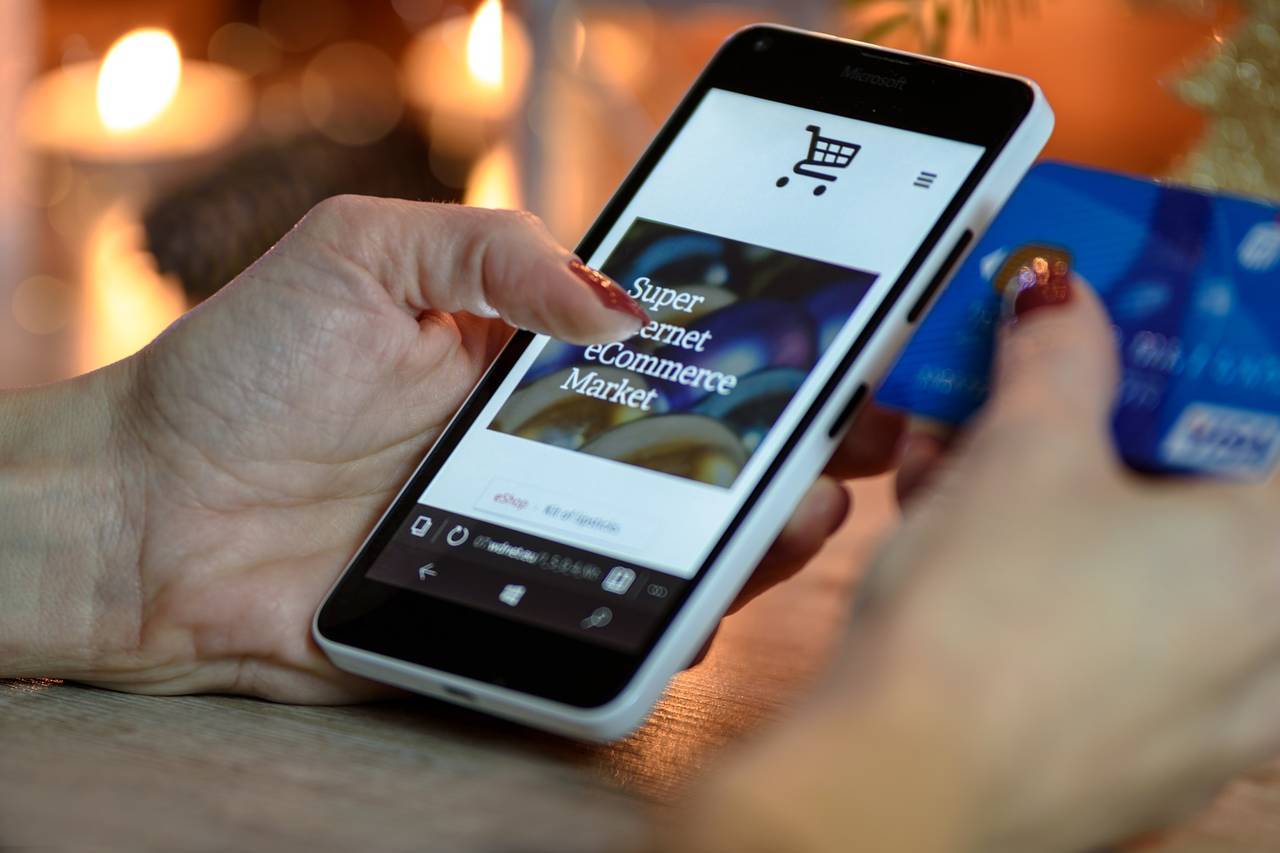