Validating A Username Using jQuery and ASP.NET Membership Provider
Earlier this year I wrote an application that allowed the user to fill in a username as part of the registration. I thought I would add a creature comfort to the form by making a non-intrusive validation of the username against the ASP.NET membership provider. My goal was pretty simple, see if the username was available and let the user know but not impede them from continuing the form. I decided to use jQuery and a simple HttpHandler.
The markup for the username line of the form contains a Label and an Input field for the username to be entered. It also contains a couple of Span tags, one for the indicator the username is being checked (usernameLoading) and one to indicate the results (usernameResult).
<
li
>
<
label
id
="lblUserName"
for
="txtUserName"
>
User Name :</
label
>
<
input
id
="txtUserName"
name
="txtUserName"
class
="fillout required"
type
="text"
maxlength
="20"
value
="<%=UserName %>"
/><
em
><
img
src
="images/required.png"
alt
="required"
/></
em
>
<
span
id
="usernameLoading"
>
<
img
src
="images/indicator.gif"
alt
="Ajax Indicator"
/></
span
>
<
span
id
="usernameResult"
>
</
span
></
li
>
The jQuery for the process assigns a ‘blur’ event handler for the txtUsername field. When a user leaves the field the blur event handler will fire. A function definition is defined in the handler that first displays the spinning Ajax indicator, then posts the value of the txtuserName field to the checkusername.ashx HttpHandler. The post method also defines a function to handle the handler’s response which fades the usernameResult Span result out if its already displayed. It then calls a custom function 400ms later, finishAjax(…).
$(function
() {$('#usernameLoading'
).hide();$('#txtUserName'
).blur(function
() { $('#usernameLoading'
).show(); $.post("checkusername.ashx"
, { username: $('#txtUserName'
).val() },function
(response) { $('#usernameResult'
).fadeOut(); setTimeout("finishAjax('usernameResult', '"
+ escape(response) +"')"
, 400); });return
false
;});function
finishAjax(id, response) { $('#usernameLoading'
).hide(); $('#'
+ id).html(unescape(response)); $('#'
+ id).fadeIn();}//finishAjax
The finishAjax function hides the Ajax processing image, sets the html of the usernameResult Span and fades it in.
The checkusername.ashx contains the code to see if the username is available. You could implement any sort of code you want in this method to perform validation on the username, password or just about anything else. In this case it simply checks to see if a user exists for the username, if so it returns some HTML to indicate the useraname is Unavailable, otherwise it lets them know it is available.
Public
Sub
ProcessRequest(ByVal
contextAs
HttpContext)Implements
IHttpHandler.ProcessRequest context.Response.ContentType ="text/plain"
Dim
muAs
MembershipUser = Membership.GetUser(context.Request("username"
))If
Not
IsNothing(mu)Then
context.Response.Write("<span style='
"color:#f00"
">Username Unavailable</span>"
)Else
context.Response.Write("<span style='
"color:#0c0"
">Username Available</span>"
)End
If
context.Response.Flush() context.Response.End
()End
Sub
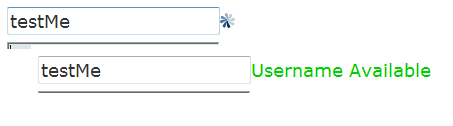
When the handler returns the resulting HTML the progress indicator is faded out and the result is faded into view. Now the user knows their username is valid and can continue with the form or choose a new username. This technique can be used to do just about any sort of client-side/server-side validation without impeding the user’s progress.