How to Use the Web Share API to Trigger the Native Dialog to Share Content & Pull Quotes
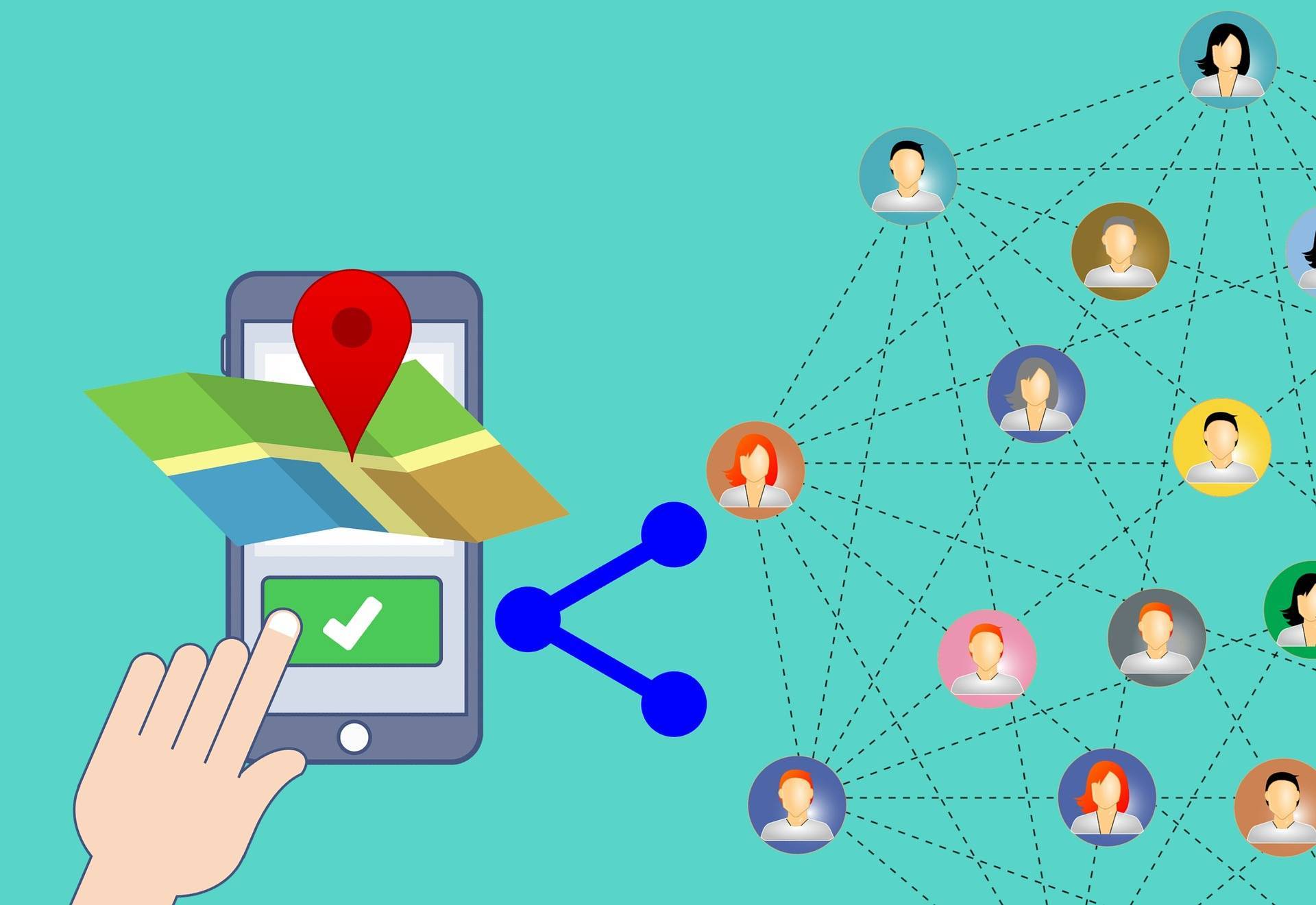
Let's face it, we all get a little rush when someone shares our content to their social media audience.
But sharing a web page to social media is complicated, right?
No!
The Web Share API brings native content sharing to the web, a feature previously limited to native apps to websites. Now you can offer a native way to share links, text and other content from a web page to the user's desired target. Targets can be social media platforms, SMS, email or other registered share targets.
You will see how the Web Share API works and how to create a fallback option for browsers not yet supporting this API.
What is Web Sharing?
There are many ways websites have tried to encourage visitors to share the page on social media. Many sites use WordPress Social share plugins or inject scripts supplied by different social media platforms.
The problem with these scripts is they inject lots of excessive JavaScript that add little value and
How do you use link sharing?
slow your page speed down. They also limit choice to the website, not the user.Most browsers, like Chrome for Android do have a native share option available, but it requires the user to dig through the browser settings menu and does not provide flexibility over what gets shared. You will also find it in Edge and Chrome desktop browsers.
You are limited to just the current URL. Plus when a progressive web app is displayed using full screen or standalone modes the browser's menu is not available.
There have been several attempts to make sharing content available to websites, such as Web Activities and Web Slices. Oh, slices I remember when they were unveiled at Mix so long ago...
Native apps have long had an advantage by offering platform share integration. This is where you can add a button to the app and it will launch a native component displaying a dialog or 'shelf' of possible share targets.
Can I Use The Web Share API?
Now you don't need to worry about adding a series of share buttons for Twitter, Facebook, Pinterest, Instagram or even SMS to your code. You can add a single action button to the page to trigger all share targets the user has registered.
The only problem with the current state of the Web Share API is being limited to Edge, Chrome and Safari. But let's face it, that covers the vast majority of users today.
How to Share a Web Page or Web Site?
You may be wondering how do you use link sharing? or How do I enable link sharing?
The Web Share API is pretty simple. Like most modern web platform APIs it requires the page use HTTPS for security. It is also uses promises.
I will show you how to share a link to a page and pull quotes from articles.
There are requirements before the API is available and can be used:
- HTTPS
- Can only be invoked in response to a user action, such as a button click
- Can share any URL or text
- always feature-detect before invoking the API
The Web Share API you should feature detected before use, so you wont throw any unwanted exceptions.
//check is the share object is a member of the navigator
var webShare = 'share' in navigator;
The share API is a single method, 'share'. It returned or resolves a Promise and accepts a single object as its parameter.
The object can have any combination of properties: title, text and url. It must contain at least one of these parameters, but all are optional.
The browser or user agent is responsible for determining what a valid share target is and how to transform the share object into a format suitable for the share target.
If the user aborts the share operation the share promise rejects with a DOMException, AbortError.
Right now the Web Share API is limited to Chrome and Safari on Android and iOS, but you will find it available in Chrome desktop.
Example Web Share Implementation
I have created a Web Share helper library to handle a common share button and share quotes. It also has a simple fallback mechanism for browsers without support.
For the past few years my blog posts have featured a row of social sharing buttons at the bottom of the page. These buttons are hyperlinks referencing a URL using each social network's built-in sharing format.
I won't go into each network's URL format, but you generally use a queryString with a collection of parameters mapping to fields the network uses. This eliminates slow JavaScript code so your page speed is on point.
Notice how the button list uses the Bootstrap 'invisible' class?
This keeps the list hidden until the share helper library has a chance to determine if the fallback or share button should be displayed.
When the Web Share API is supported a single share button is displayed.
The share button also uses the 'invisible' class and is removed if the Web Share API is available.
The share button has some 'share' attributes that map to the Share API method properties: title, text and url. The library reads these attributes and passes the values to the share method.
To invoke the Share API it is a call to the 'share' method. Pass an object with at least one of the possible fields.
if ( webShare ) {
var share = {
title: self.getAttributeValue( target, "share-title" ),
text: self.getAttributeValue( target, "share-text" ),
url: self.getAttributeValue( target, "share-url" ) };
navigator.share( share )
.then( function () {
console.log( 'Successful share' ) } )
.catch( function ( error ) {
console.log( 'Error sharing', error )
}
);
}
When invoked the native share dialog is displayed with available share targets.
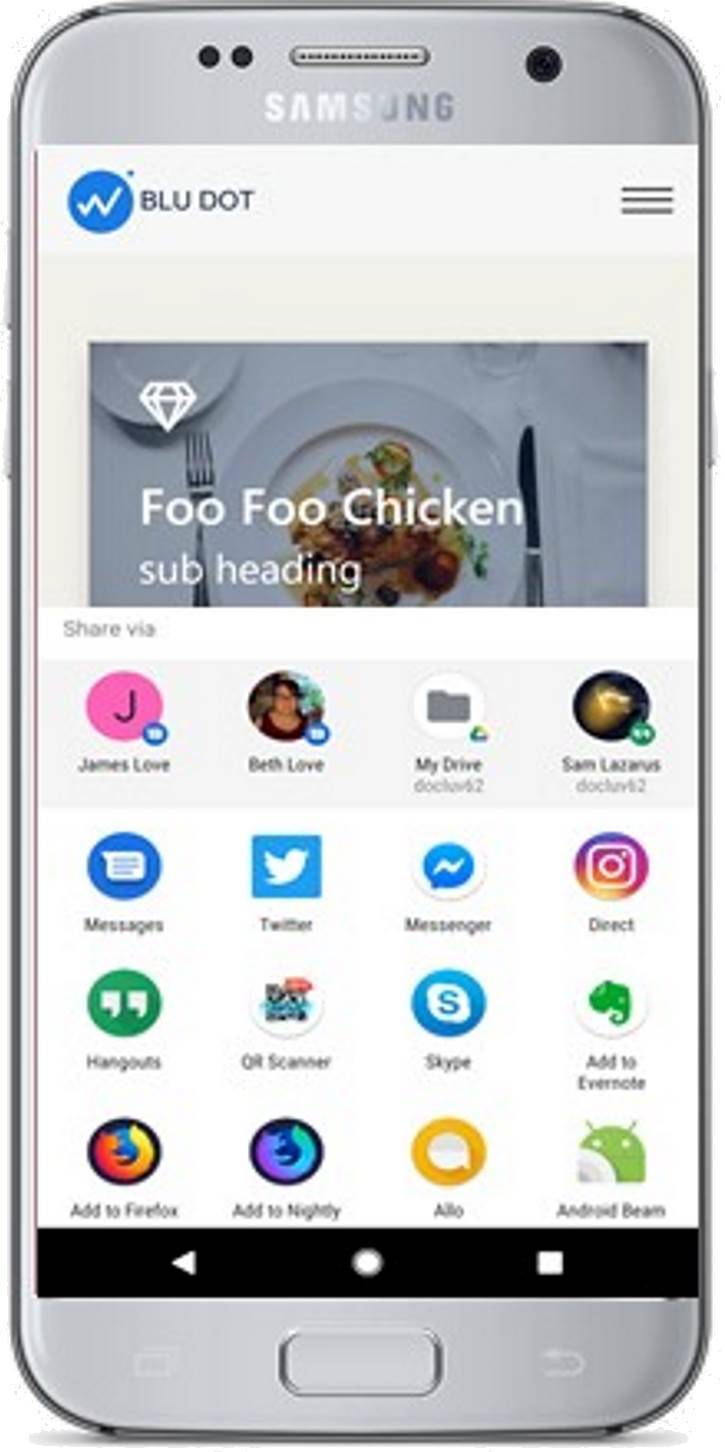
Sharing Article Pull Quotes
Share buttons to share an article are helpful, but you can also sculpt specific, important quotes from your content without a single button.
By doing this you are making it super easy for readers to share important content from your article with their tribes.
My Share library automatically looks for elements using the 'share-quote' class. When it finds these targets it binds a method in response to the user clicking or tapping the target.
The quote target should use the same attributes as the share button example above.
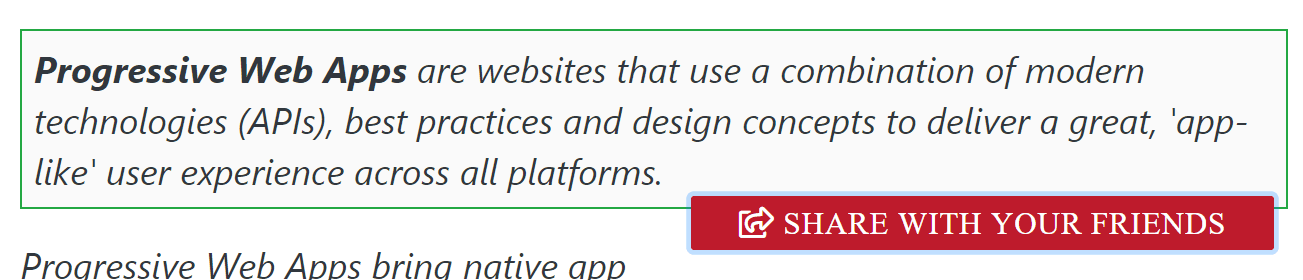
The library automatically finds share buttons and quote targets. You just need to make sure the markup is available before the script executes.
Summary
The Web Share API is a modern web platform feature that makes a common practice native to the web. Now visitors can share your content easier across social networks, SMS and registered target apps.
My little web share library makes it a little easier for you to use this API with just simple HTML around your content for quotes and to replace messy third party scripts. It also provides a simple way to display fallback share options.
Right now the Web Share API is only available in Chrome and Safari for mobile platforms. But is present in Chrome Desktop and will launch the native share dialog. Once share targets are available they are listed in the native share dialog.